Recently I’ve been working on the WPF application that extensively uses Caliburn.Micro framework for MVVM pattern. MVVM
usually force you to write many properties in the ViewModel
. Those properties should typically notify View
about their values changes – to do that every ViewModel
has to implement INotifyPropertyChanged interface and in the setters of the properties we need to rise NotifyPropertyChanged
event, for example like that:
public class SelectTagsViewModel : Screen
{
public event PropertyChangedEventHandler PropertyChanged;
private string _property;
public string Property
{
get { return _property; }
set
{
_property = value;
NotifyPropertyChanged("Property");
}
}
private void NotifyPropertyChanged(string name)
{
if (PropertyChanged != null)
{
PropertyChanged(this, new PropertyChangedEventArgs(name));
}
}
}
In Caliburn.Mirco
your ViewModel
can inherit from PropertyChangedBase
class (or other classes that internally inherit from PropertyChangedBase
class – like Screen
, Conductor
and so on) and then in properties you can use NotifyOfPropertyChange
method:
private string _property;
public string Property
{
get { return _property; }
set
{
_property = value;
NotifyOfPropertyChange(() => Property);
}
}
Because in my project I have to write many properites with NotifyOfPropertyChange
method in their setters, I’ve decided that I write Resharper Live Template for this purpose. To do this you should go to the ‘Resharper‘ menu in Visual Studio, then ‘Live Templates‘ and there you should select ‘New Template‘ button. After this you will get the window like this one:
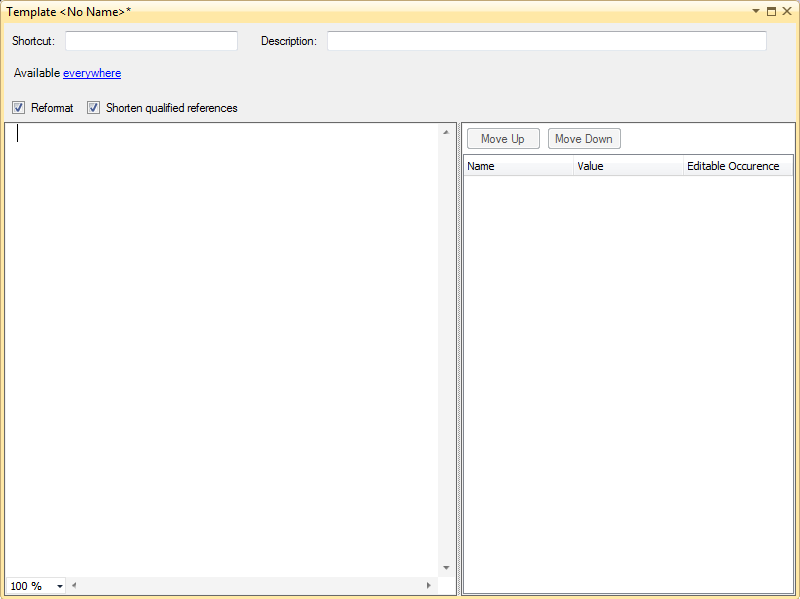
In the left part you can write the code of the snipped. In my case it was:
private $TYPE$ _$PRIVATEMEMBER$;
public $TYPE$ $PROPERTYNAME$
{
get { return _$PRIVATEMEMBER$; }
set
{
_$PRIVATEMEMBER$ = value;
NotifyOfPropertyChange(() => $PROPERTYNAME$);
}
}
where:
$TYPE$
– the type of the property$PRIVATEMEMBER$
– the name of the private field$PROPERTYNAME$
– the name of the property
The most tricky part of this template is setting properties of the template in the right pane of the window. This should be done in this way:
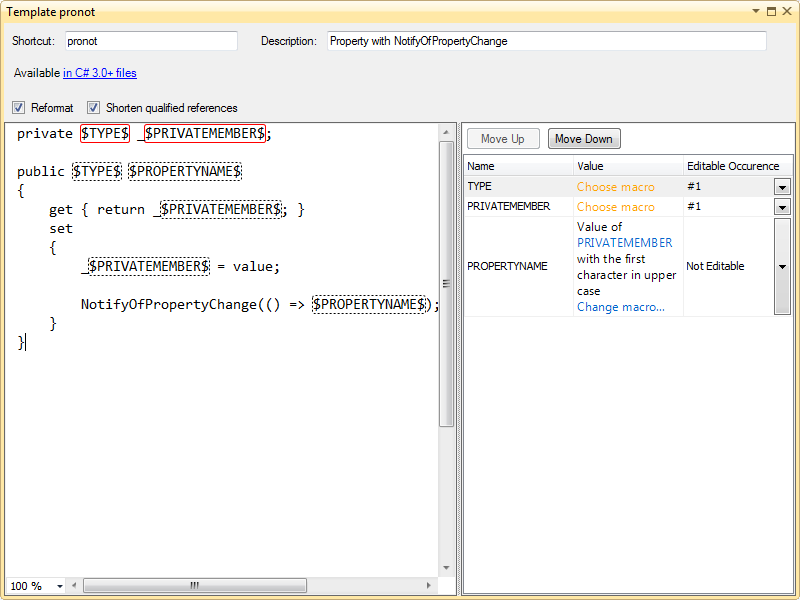
- In the
Editable Occurrence
column we specify the order of defining elements of the template – first the type and the name of the field that will be used for this property. - In the
Value
column we can set how the value of the template field will be calculated – the value of$PROPERTYNAME$
property is set as name of the private field with first character as upper case.
The last thing to do is to set a shortcut for this snippet (in this case: propnot
) and the description. And this is it, after saving you have a new live template that can be use in code editor by simply writing “propnot“. If you do that you will get something like this in Visual Studio:
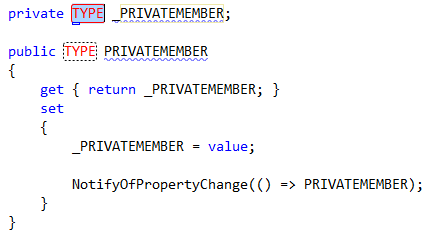